Introduction
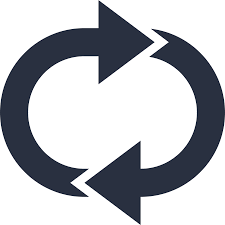
Now that you’re familiar with the basics of Java, it’s time to learn about loops! Loops are a key feature in programming, allowing you to execute a block of code multiple times. In this lesson, we’ll focus on two common types of loops in Java: for and while loops.
What is a For Loop?
A for
loop is great when you know exactly how many times you want to repeat a task. Here’s the structure:
for (initialization; condition; update) {
// Code to execute
}
Example: Printing Numbers from 1 to 5
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
Output:1 2 3 4 5
What is a For Loop?
A while
loop is ideal when the number of iterations depends on a condition being true. Here’s how it works:
while (condition) {
// Code to execute
}
Example: Printing Numbers from 1 to 5
int i = 1;
while (i <= 5) {
System.out.println(i);
i++;
}
Output:1 2 3 4 5
For Loop vs. While Loop
For Loop | While Loop |
---|---|
Use when iterations are known. | Use when iterations depend on a condition. |
Initialization, condition, and update are in one line. | Initialization and update are written separately. |
Practice Activity
- Watch the video tutorial below and finish the pop quiz inside.
- You are ready! now take our ultimate final exam to prove yourself!
- Independently use the Java language to print a pyramid using what we have learned in these two lessons (print syntax, variables, for/while loop), using the character “*” and the number of pyramid levels to be three or more.
Java Boot Camp Ultimate Final Exam
* The contents in this final exam is made with assistance of ChatGPT 4o
Grade scale:
- 11/11: A+
- 8/11: A
- 6/11: B
- 4/11: C
- 0-3/11: D
Send the output pyramid visual pattern in the comments section and ask questions if you have any.
References
“Quiz questions for Java on the topic of for/while loop, print and variables” prompt. ChatGPT, 29 Nov. version, OpenAI, 3 Dec. 2024, chat.openai.com/chat.
Recent Comments