Introduction
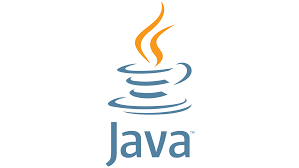
Welcome to your first step in learning Java! In this lesson, we’ll cover two fundamental building blocks of Java programming: print statements and variables. These concepts are essential for displaying outputs and storing data in your programs. If you’re ready, let’s dive in!
Our Study Tool: W3School
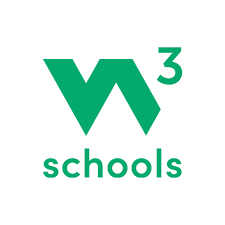
For this journey, we’re using the beginner-friendly online compiler available on W3Schools to guide us. Let’s dive in!
What is a Print Statement?
In Java, the System.out.println()
method is used to display messages or data on the screen. It’s a simple but powerful way to see the results of your code. Here’s an example:
System.out.println("Hello, World!");
Output:Hello, World!
You can also combine text and variables to make your outputs dynamic. For example:
int age = 25;
System.out.println("Your age is: " + age);
Output:Your age is: 25
What Are Variables?
Variables are containers for storing data. Each variable has:
- Type (e.g.,
int
,String
). - Name (what you call the variable).
- Value (the data it holds).
Example: Declaring and Initializing Variables
int age = 25; // Integer type
String name = "Alice"; // String type
You can use these variables in calculations or to store user input. For example:
int a = 5;
int b = 10;
int sum = a + b;
System.out.println("Sum: " + sum);
Output:Sum: 15
Practice Activity
- Watch the video tutorial below and finish the pop quiz inside.
- Create a program on W3Schools that calculates and prints the area of a rectangle. (Hint: Use
length * width
.)
If you have any thoughts or questions about the lecture, please feel free to comment below.
Recent Comments